In this post, I'll show you how to draw simple graphics with this API. HTML 5 Canvas is an HTML tag that you can embed inside an HTML document. Here's a sample html document containing the canvas element:
<!DOCTYPE HTML> <html> <body> <canvas id="canvasId" width="578" height="200"> </canvas> </body> </html>
With the element declared in the html, you can then create a JavaScript script to retrieve that canvas element and create a 2D or 3D drawing context like this:
window.onload = function(){ var canvas = document.getElementById("canvasId"); var context = canvas.getContext("2d"); // draw anything here };
Drawing A Line
To draw a line, you need first to set the lineWidth (line thickness) and strokeStyle (line color). Then you need to tell the drawing context to move from the starting point to the ending point in pixels like so:// set the line thickness to 5 pixels context.lineWidth = 5; // set the line color to red context.strokeStyle = "red"; // position the drawing cursor context.moveTo(40, canvas.height - 40); // draw the line context.lineTo(canvas.width - 40, 40); // make the line visible with the stroke function: context.stroke(); };
Here's the screenshot of the drawn line as being displayed in FireFox:
Drawing An Arc
To draw an arc, you need first to set the lineWidth (line thickness) and strokeStyle (line color). Then you need call the arc function from the drawing context specifying the center location, radius, starting angle, ending angle and draw direction like so:context.arc(centerX,centerY, radius, startingAngle, endingAngle,counterclockwise);
context.lineWidth = 15; context.strokeStyle = "red"; // line color context.arc(canvas.width / 2, canvas.height / 2 + 40, 80, 1.1 * Math.PI, 1.9 * Math.PI, false); context.stroke(); };
Here's the screenshot of the drawn arc as being displayed in FireFox:
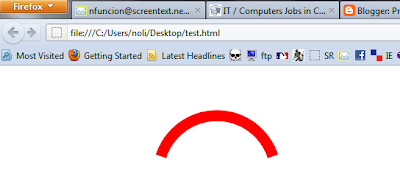
Drawing A Rectangle
To draw a rectangle, you need to call the rect function of the drawing context specifying the center location x and y,width and height. Then you need to specify the fillStyle (rectangle's fill color), lineWidth (line thickness) and strokeStyle (line color). You need to call fill() and stroke() to execute the fill and stroke drawing respectively like so:context.rect(x,y,width,height);
context.rect(canvas.width / 2 - 100, canvas.height / 2 - 50, 200, 100); context.fillStyle = "green"; context.fill(); context.lineWidth = 5; context.strokeStyle = "red"; context.stroke(); };
Here's the screenshot of the drawn rectangle as being displayed in FireFox:
Drawing A Circle
To draw a circle, you can use the arc function above with the starting angle set to zero and the ending angle set to 2PI like so:context.arc(canvas.width / 2, canvas.height / 2, 70, 0, 2 * Math.PI, false); context.fillStyle = "green"; context.fill(); context.lineWidth = 5; context.strokeStyle = "red"; context.stroke(); }; };
Here's the screenshot of the drawn circle as being displayed in FireFox:
Drawing An Image
Canvas can be used to draw an image based on an image file from disk. All you need to do is to create an image object, set the source of the image to an image from file. Then you need to create an onload handler for the image that will trigger once the image is loaded. Inside the handler, you tell the context to draw the image and the location x and y in pixels.
var imageObj = new Image(); imageObj.onload = function(){ var destX = canvas.width / 2 - this.width / 2; var destY = canvas.height / 2 - this.height / 2; context.drawImage(this, destX, destY); }; imageObj.src = "pitchlocations.jpg";
Here's the screenshot of the drawn image with dynamically generated balls from my project as being displayed in FireFox:
This API is rockin' dude and we're only scratching the surface here. There's still a lot to learn! This topic is so broad it can easily fill a book. Just find other documentations to see how the other graphics are created, and how animations, videos and the fantastic 3D rendering are done. Hope you learn something from here.